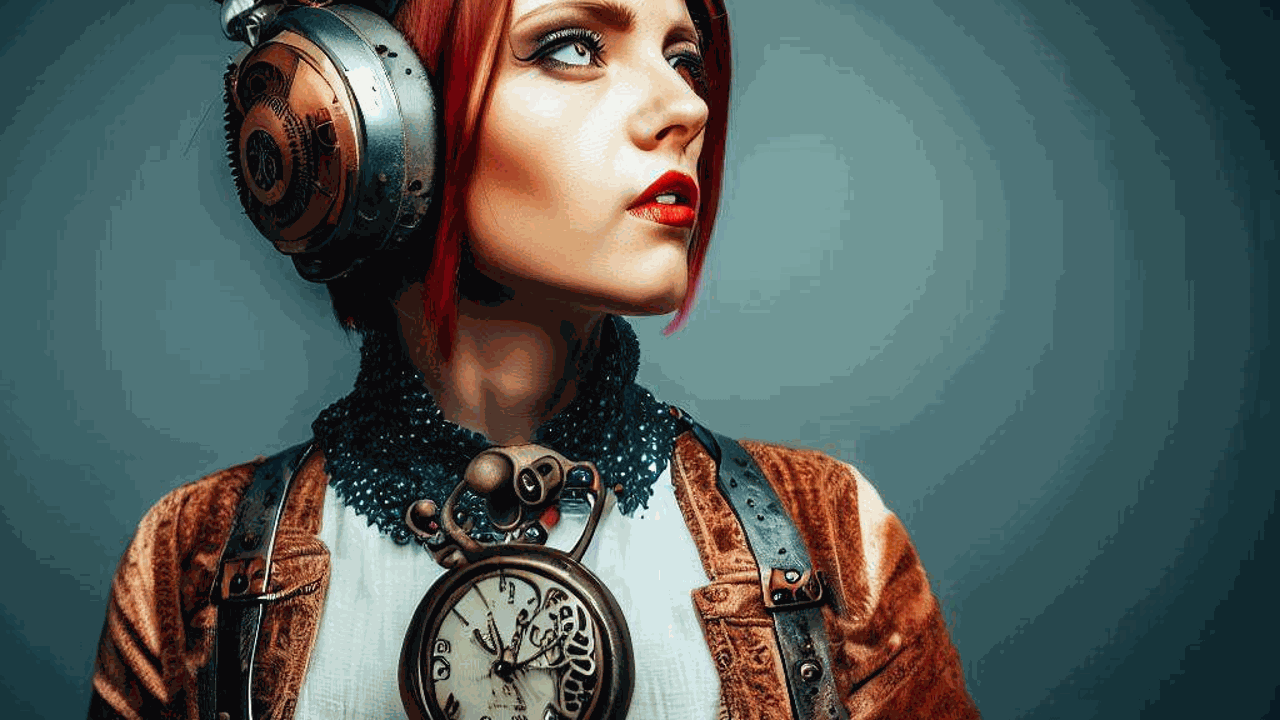
Multiple Time Series Forecasting With N-BEATS In Python
Imagine having a robust forecasting solution capable of handling multiple time series data without relying on complex feature engineering. That’s where N-BEATS comes in! In this tutorial, I’ll break down its inner workings, walk you through the process of installing and configuring NeuralForecast to train an N-BEATS model in Python, and show you how to effectively prepare and split your time series data. Furthermore, we’ll explore hyperparameter tuning with Optuna....